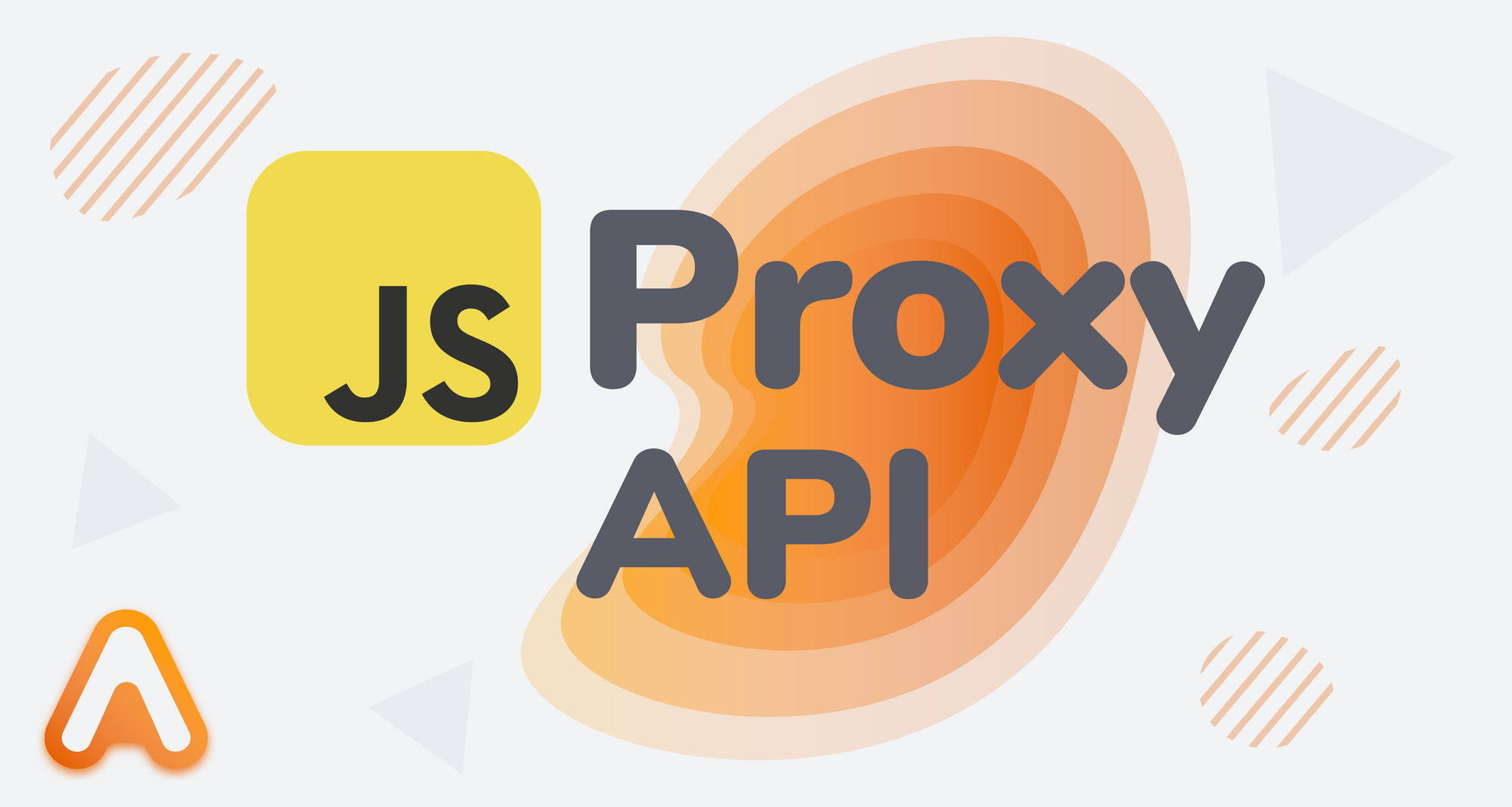
JavaScript Proxy Object Explained
JavaScript has had setters and getters support for a long time. They utilize a simple syntax with set
and get
keywords to intercept the object’s property access and value change operations.
const obj = {
propValue: 1,
get prop() {
console.log("Retrieving property prop");
return this.propValue;
},
set prop(value) {
console.log("Setting property prop to", value);
this.propValue = value;
}
};
obj.prop; // 1 | [in console] Retrieving property prop
obj.prop = 2; // [in console] Setting property prop to 2
However, setters/getters have multiple drawbacks:
- They limit you only to set and get operations (obviously).
- They don’t work together with data entry of the same key i.e. “usual” property.
- They aren’t dynamic and have to be explicitly applied to each property during object declaration, with static
[Object.defineProperty()](/secrets-of-the-javascript-object-api)
method, or by using a computed value (newer browsers only).
// ...
Object.defineProperty(obj, "anotherProp", {
get() {
/* Do something on get */
},
set(value) {
/*Do something on set */
}
});
So, setters and getters aren’t very suitable for observing entire objects or performing very simple operations. And that’s why ECMAScript 6 (ES6) introduced the Proxy object.
Proxy
Proxy is a built-in JS object that can be used to intercept and alter the behavior of different object-related operations.
const originalObj = { prop: 1, anotherProp: "value" };
const proxyObj = new Proxy(originalObj, {
get(obj, prop) {
console.log("Retrieving property", prop);
return obj[prop];
},
set(obj, prop, value) {
console.log("Setting property", prop, "to", value);
obj[prop] = value;
return true;
}
});
originalObj.prop; // 1
originalObj.prop = 2;
proxyObj.prop; // 2 | [in console] Retrieving property "prop"
proxyObj.anotherProp = "new value"; // [in console] Setting property "anotherProp" to "new value"
Right off the bat, we can see the differences between the Proxy vs setters and getters. Not only they differ in terms of syntax (Proxies are more verbose), but also in terms of the interaction with the original object. The Proxy creates a new object for you to interact with rather than the original object, which is altered directly when working with setters/getters.
In the case of Proxy, the original object (aka target
) is used as a sort of storage. Any operation you perform on it directly affects the Proxy but doesn’t trigger any of its traps.
Proxy’s traps are simple methods that are called when the specific operation gets performed. They’re all defined on a single handler object which is then passed to the Proxy constructor. Also, they aren’t limited to the only set()
and get()
but also a few other interesting operations that you can find which you can read about in-depth on MDN documentation.
Revocable Proxies
If for any reason you’d want to be able to cancel/revoke your Proxy later, you should create it with the static [Proxy.revocable()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Proxy/revocable)
method.
// ...
const revocableProxyObj = Proxy.revocable(originalObj, {
get(obj, prop) {
/* Do something on get */
},
set(obj, prop, value) {
/*Do something on set */
}
});
const proxy = revocableProxyObj.proxy;
proxy.prop; // OK
revocable.revoke();
proxy.prop // TypeError
Instead of returning the Proxy object directly, this method returns an object containing the actual Proxy under proxy
property and an additional revoke()
method.
When called, this method invalidates the Proxy, making any future call end with a TypeError
. After that the Proxy will be automatically garbage-collected, freeing the space in memory.
Use-cases
When compared to setters/getters Proxies allow you to do a lot more. They’re a bit faster (after declaration) and more flexible, making them a perfect solution for use-cases like state management.
Proxies bring a lot of customizability to the table giving the developer control over some meta-functionalities of JS. Thus, they aren’t backward-compatible and there’s no option for any fully-compatible polyfill to exist. With that said, according to the data from Can I use…, general support looks decent, with about 93% coverage (missing IE and Safari <10).
Summary
That’s it for this article! Let me know in the comments what do you think of such a brief and to-the-point form! I hope you enjoyed it and learned something new. If so consider sharing it and following me on Twitter, Facebook or through my weekly newsletter. I’ve also got a YT channel which you might want to check out. Thanks for reading this piece and have an awesome day!
If you need
Custom Web App
I can help you get your next project, from idea to reality.